Downloading Data Programmatically From a Portal
Portals are websites created to promote data sharing within a specific research community. These websites aggregate relevant data from Synapse, and they allow users to explore data, projects, people, and organizations within their research community.
Sage Bionetworks hosts a variety of web portals for different research communities. The AD Knowledge Portal, the NF Data Portal, and the PsychENCODE Knowledge Portal are a few examples. The following guide describes how to programmatically download data discovered on a portal.
Find Files Using Explore
All entities in Synapse are automatically assigned a globally unique identifier used for reference with the format syn12345678
. Often abbreviated to “synID”, the ID of an object never changes, even if the name does. You will use a synID to locate the files you wish to download.
Search the available data files via the Explore tab in the navigation bar. The Explore section presents several ways to select data files of interest. The top of the page displays pie charts that summarize the data files based on file annotations of interest, including Assay and Tissue, among others. Selection of one of these chart segments will filter the table below to subset the set of files. Alternatively, access the filters using the facet selection boxes to the left of the table. For this example, you will download the processed data and metadata from the MC-CAA study in the Alzheimer’s Disease (AD) Knowledge Portal.
Download Files
Command Line
The Synapse command line client can be used to download all data and file annotations with a single command.
The command line client is installed with the Synapse Python client, therefore Python 3 is required to install the Synapse command line client. Login to Synapse. If working on your personal computer, you may store your credentials locally by including the --rememberMe
argument to allow automatic authentication with future Synapse interactions. This is recommended to prevent a case where you might accidentally share your password while sharing analytical code.
To login with your username and password, e.g.
synapse login -u <username> -p <password> --rememberMe
A Synapse access token is more secure than your password and is highly recommended to be used to login instead of using your password.
synapse login -p <access token> --rememberMe
From Explore Data in the portal, select the Download Options icon and Programmatic Options to visualize the command to download the data subset.
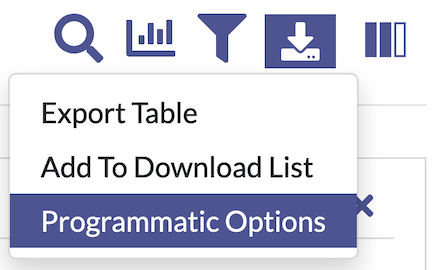
The command synapse get
with the -q
argument downloads files that meet the specified condition. In this example, all processed and metadata files from the MC-CAA study will be downloaded. Execute the following command from the directory where you would like to store the files.
synapse get -q "SELECT * FROM syn11346063 WHERE ( ( "study" = 'MC-CAA' ) AND ( "dataSubtype" = 'processed' OR "dataSubtype" = 'metadata' ) )"
Also in your working directory, you will find a SYNAPSE_TABLE_QUERY_###.csv
file that lists the annotations associated with each downloaded file. Here, you will find helpful experimental details relevant to how the data was processed. Additionally, you will find important details about the file itself including the file version number.
R
In order to download data programmatically with R, you need a list of synIDs that correspond to the files. For downloading a large set of files, we recommend using the Synapse Python client. The Python client has been optimized for multi-threaded download and will provide you with faster download speeds.
Once you have identified the files you want to download from Explore Data, use the Export Table option from Download Options. The table includes annotations associated with each downloaded file.
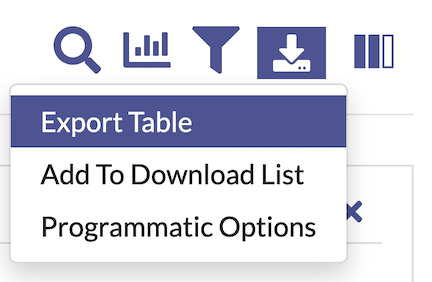
You may choose to download the file as a .csv
or .tsv
. Files are named Job-####
(where # is a long set of numbers). Move this file to your working directory to proceed with the following steps.
Install the Synapse R client synapser
to download data from Synapse. Login using your password or preferably an access token.
library(synapser)
# using password
synLogin("my_username", "password")
# OR using an access token
synLogin(authToken="token")
Read the exported table into R replacing Job-####
with the complete filename of the downloaded table. Create a directory to store files and download data using synGet
. If downloadLocation
is not specified, the files are downloaded to a hidden directory called ~/.synapseCache
.
exported_table <- read.csv("Job-####.csv")
dir.create("files")
lapply(exported_table$id, synGet, downloadLocation = "./files")
The annotations in exported_table
include experimental details relevant to how the data was processed.
Python
In order to download data programmatically, you need a list of synIDs that correspond to the files.
Once you have identified the files you want to download from Explore Data, use the Export Table option from Download Options. The table includes annotations associated with each downloaded file.
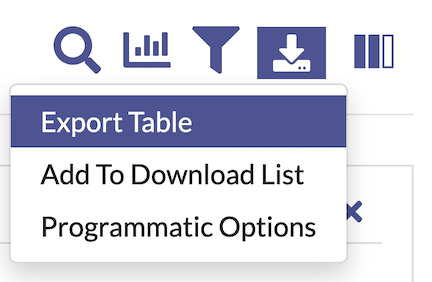
You may choose to download the file as a .csv
or .tsv
. Files are named Job-####
, where # includes a long set of numbers. Move this file to your working directory to proceed with the following steps.
Install the Synapse Python client synapseclient
to download data from Synapse, the pandas
library to read a csv file and the os
module to make a directory. Login to Synapse using your password or preferably an access token.
import synapseclient, pandas, os
syn = synapseclient.Synapse()
# login using a password
syn.login('my_username', 'password')
# OR using an access token
syn.login(authToken='token')
Read the exported table into Python replacing Job-####
with the complete filename of the downloaded table. Create a directory to store files and download data using syn.get
. If downloadLocation
is not specified, the files are downloaded to a hidden directory called ~/.synapseCache
.
exported_table = pandas.read_csv("Job-####.csv")
os.mkdir("files")
[syn.get(x, downloadLocation = "./files") for x in exported_table.id]
The annotations in exported_table
include experimental details relevant to how the data was processed.
Need More Help? Ask a question in the Synapse Help Forum. Your feedback is key to improving our documentation, so contact us if something is unclear or open an issue.